ECMAScript Rocks - EdgeTTS. Who Needs Python?
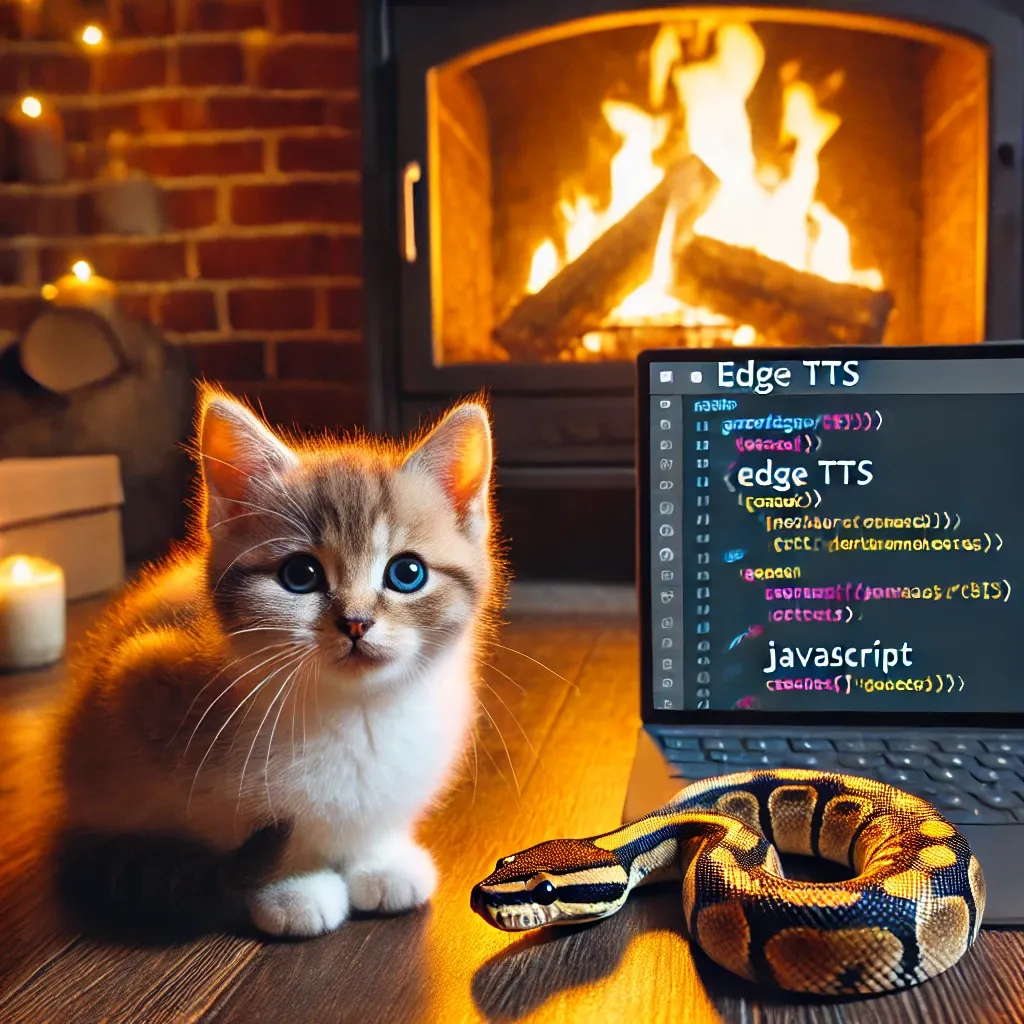
Introduction
Edge-TTS is one of those fascinating technologies with vague license and questionable legitimacy—yet it just performs. I Presume you've used the Edge-TTS Python package. In that case, you might already understand it taps into Microsoft's Bing Text-to-Speech API—the same API powering read-aloud functionality in Microsoft Edge.
But here's the real kicker: Edge-TTS relies on a TRUSTED_CLIENT_TOKEN floating around in the wild, frankly used in numerous repositories and browser extensions. Who disseminated it? Is it lawful to use? No one is sure yet, yet it works, and that's what matters, providing it's legal. Surely someone would tell it? If not, this is also free regarding translation and making it complete, giving it English text and translating it to another language and making it speak, simple as...
🔗 Check out the live demo here!
Breaking It Down: How Edge-TTS Works
At its core, Edge-TTS connects to Microsoft's speech synthesis API using WebSockets, sending SSML (Speech Synthesis Markup Language) requests to generate speech dynamically. Here's what makes it tick:
- WebSockets: Establishes a real-time connection to the Bing API.
- Trusted Client Token: The magic key that allows Edge-TTS to function. No one knows who officially issued it, but it's widely used.
- Sec-MS-GEC Header: A SHA-256 hash that ensures timestamp validity.
- SSML Input: This tells the TTS engine which voice to use, pitch, speed, and pauses.
- Audio Stream Output: The API sends back real-time MP3 audio, which plays directly in the browser.
Edge-TTS in the Wild
This isn't just some obscure API locked inside Microsoft Edge. It's available as:
- A Python package (pip install edge-tts).
- A Google Chrome extension that enables text-to-speech on webpages.
- A standalone JavaScript implementation, which we're showing off today.
But… Is It Legal?
Now comes the awkward question: is using Edge-TTS outside of Microsoft Edge legal?
The edge-tts Python package and several browser extensions openly use this API. Although Microsoft has never publicly documented the API for third-party use, it remains accessible.
- No API keys beyond the likely token are needed.
- There are no official rate limits.
- No clear terms of service.
This raises a big question: Does Microsoft allow this, or have they just not caught on? Until they do, it works, so let's use it and do not let them say we have not told you so.
Here It Is, Working in JavaScript!
We've taken Edge-TTS and built a pure JavaScript solution. No Python, no dependencies—just a browser, WebSockets, and JavaScript
📝 View the working source code
The basis of which is below. adapt and learn while taking care never to change a header, for should you do that the whole code breaks. Headers are IMPORTANT!
<script>
/*
(C) 2025 AKADATA LIMITED - 28 Feb 2025 - Andrew Smalley
Based on the Python edge-tts module and Azure EdgeTTS service.
This code demonstrates a JavaScript implementation inspired by the Python edge-tts module.
It connects to the Azure EdgeTTS service via WebSocket, sending properly formatted headers and SSML
to synthesize speech from text. The headers in the requests are critical for proper routing and
processing by the service—do not edit these headers unless you know exactly what you're doing.
How can this be improved?
- Error handling can be further refined for robustness.
- The code could be modularized further by separating concerns (e.g., a separate module for header construction).
- Additional customization of SSML parameters (e.g., voice effects) can be provided.
- Support for additional languages and voices could be integrated.
*/
/**
* DRM class handles token generation and timestamp calculations required for authentication.
* It mirrors functionality from the Python edge-tts module by creating secure tokens
* using SHA-256 hashing.
*/
class DRM {
static WIN_EPOCH = 11644473600; // Windows epoch offset in seconds.
static S_TO_NS = 1e9; // Conversion factor from seconds to nanoseconds.
static TRUSTED_CLIENT_TOKEN = "6A5AA1D4EAFF4E9FB37E23D68491D6F4"; // Fixed token for service authentication.
static clockSkewSeconds = 0; // Allows adjustment for client-server clock skew.
/**
* Adjusts the internal clock skew.
* @param {number} skewSeconds - Number of seconds to adjust.
*/
static adjustClockSkew(skewSeconds) {
DRM.clockSkewSeconds += skewSeconds;
}
/**
* Returns the current Unix timestamp adjusted for any clock skew.
* @returns {number} Adjusted Unix timestamp.
*/
static getUnixTimestamp() {
return Math.floor(Date.now() / 1000) + DRM.clockSkewSeconds;
}
/**
* Generates a secure message digest for authentication.
* The digest is calculated using a combination of the timestamp and the trusted client token.
* @returns {Promise<string>} A promise that resolves to the uppercase hexadecimal digest.
*/
static async generateSecMsGec() {
let ticks = DRM.getUnixTimestamp() + DRM.WIN_EPOCH;
// Round down to nearest multiple of 300 seconds.
ticks -= ticks % 300;
// Scale ticks; note: dividing by 100 adjusts the scale for the API.
ticks *= DRM.S_TO_NS / 100;
const strToHash = `${Math.floor(ticks)}${DRM.TRUSTED_CLIENT_TOKEN}`;
const hashBuffer = await crypto.subtle.digest("SHA-256", new TextEncoder().encode(strToHash));
return Array.from(new Uint8Array(hashBuffer))
.map(b => b.toString(16).padStart(2, "0"))
.join("")
.toUpperCase();
}
}
/**
* EdgeTTS class provides the core functionality for interacting with the Azure EdgeTTS service.
* It is inspired by the Python edge-tts module, but implemented in JavaScript to run directly in the browser.
*/
class EdgeTTS {
// Base URL for the EdgeTTS service.
static BASE_URL = "speech.platform.bing.com/consumer/speech/synthesize/readaloud";
// URL to fetch the list of available voices. Do not modify the headers used in these requests.
static VOICE_LIST_URL = `https://${EdgeTTS.BASE_URL}/voices/list?trustedclienttoken=${DRM.TRUSTED_CLIENT_TOKEN}`;
// Default voice to use if no preference is specified.
static DEFAULT_VOICE = "en-GB-LibbyNeural";
constructor() {
this.voice = EdgeTTS.DEFAULT_VOICE;
this.voiceList = [];
this.ws = null;
this.audioChunks = [];
}
/**
* Fetches available voices from the EdgeTTS service.
* The headers specify that the response should be in JSON format.
* Note: Do not edit the "Accept" header if you want this to work.
* @returns {Promise<Array>} A promise that resolves to an array of voice objects.
*/
async fetchVoices() {
try {
const response = await fetch(EdgeTTS.VOICE_LIST_URL, {
headers: {
"Accept": "application/json"
}
});
if (!response.ok) throw new Error(`HTTP Error: ${response.status}`);
const voices = await response.json();
// Cache voices in localStorage for faster access.
localStorage.setItem("edge_tts_voices", JSON.stringify(voices));
this.voiceList = voices;
return voices;
} catch (error) {
console.error("Failed to fetch voices:", error);
return [];
}
}
/**
* Determines the best available voice.
* It first checks for the preferred default voice and falls back to the best alternative.
* @returns {Promise<string>} A promise that resolves to the chosen voice's short name.
*/
async getBestVoice() {
let voices = JSON.parse(localStorage.getItem("edge_tts_voices")) || [];
if (!voices.length) voices = await this.fetchVoices();
const preferredVoice = voices.find(v => v.ShortName === EdgeTTS.DEFAULT_VOICE);
if (preferredVoice) return EdgeTTS.DEFAULT_VOICE;
const fallbackVoice = voices.find(v => v.Locale.startsWith("en-GB")) || voices[0];
return fallbackVoice.ShortName;
}
/**
* Establishes a WebSocket connection to the EdgeTTS service.
* It generates the required secure digest and connection ID.
* @returns {Promise<void>} Resolves when the connection is successfully established.
*/
async connect() {
const secMsGec = await DRM.generateSecMsGec();
const connectionId = crypto.randomUUID().replace(/-/g, "");
const url = `wss://${EdgeTTS.BASE_URL}/edge/v1?TrustedClientToken=${DRM.TRUSTED_CLIENT_TOKEN}&Sec-MS-GEC=${secMsGec}&Sec-MS-GEC-Version=1&ConnectionId=${connectionId}`;
return new Promise((resolve, reject) => {
this.ws = new WebSocket(url);
this.ws.binaryType = "arraybuffer"; // Ensures binary audio data is correctly handled.
this.ws.onopen = () => {
console.log("WebSocket connected.");
resolve();
};
this.ws.onerror = (error) => {
console.error("WebSocket Error:", error);
reject(error);
};
this.ws.onclose = (event) => {
console.warn(`WebSocket closed. Code: ${event.code}, Reason: ${event.reason}`);
};
this.ws.onmessage = (event) => this.processMessage(event);
});
}
/**
* Sends the audio configuration to the EdgeTTS service.
* The headers in this message (e.g., Content-Type, Path) are critical—do not edit them if you want this to work.
*/
async sendAudioConfig() {
const requestId = crypto.randomUUID().replace(/-/g, "");
const config = [
`X-RequestId:${requestId}`,
"Content-Type:application/json; charset=utf-8",
"Path:speech.config",
"",
'{"context":{"synthesis":{"audio":{"metadataoptions":{"sentenceBoundaryEnabled":"true","wordBoundaryEnabled":"false"},"outputFormat":"audio-24khz-96kbitrate-mono-mp3"}}}}'
].join("\r\n");
this.ws.send(config);
}
/**
* Sends an SSML (Speech Synthesis Markup Language) request to synthesize the given text.
* The headers used here (Content-Type, X-Timestamp, Path) must not be altered.
* @param {string} text - The text to synthesize.
*/
async sendSSML(text) {
const requestId = crypto.randomUUID().replace(/-/g, "");
const timestamp = new Date().toUTCString();
const ssml = this.generateSSML(text);
const message = [
`X-RequestId:${requestId}`,
"Content-Type:application/ssml+xml",
`X-Timestamp:${timestamp}Z`,
"Path:ssml",
"",
ssml
].join("\r\n");
console.log("Sending SSML request...");
this.ws.send(message);
}
/**
* Generates SSML markup based on the provided text.
* The SSML defines the voice and prosody settings (pitch, rate, volume).
* @param {string} text - The text to be synthesized.
* @returns {string} The SSML string.
*/
generateSSML(text) {
return `<speak version='1.0' xmlns='http://www.w3.org/2001/10/synthesis' xml:lang='en-GB'>
<voice name='${this.voice}'>
<prosody pitch='-2Hz' rate='+1%' volume='+0%'>${text}</prosody>
</voice>
</speak>`;
}
/**
* Processes messages received from the WebSocket.
* It distinguishes between string messages and binary audio data.
* @param {MessageEvent} event - The WebSocket message event.
*/
processMessage(event) {
// If the message is a string, check for end-of-transmission.
if (typeof event.data === "string") {
if (event.data.includes("Path:turn.end")) {
this.finishAudioProcessing();
}
}
// If the message is binary data (ArrayBuffer), store it as an audio chunk.
else if (event.data instanceof ArrayBuffer) {
this.audioChunks.push(event.data);
}
// If the message is a Blob, determine if it's audio data or text.
else if (event.data instanceof Blob) {
if (!event.data.type || event.data.type.startsWith("audio") || event.data.type === "application/octet-stream") {
event.data.arrayBuffer().then(buffer => {
this.audioChunks.push(buffer);
}).catch(err => {
console.error("Failed to convert Blob to ArrayBuffer:", err);
});
} else {
event.data.text().then(text => {
if (text.includes("Path:turn.end")) {
console.log("Audio transmission complete.");
this.finishAudioProcessing();
}
}).catch(err => {
console.error("Failed to decode Blob text:", err);
});
}
} else {
console.warn("Unknown message type:", event.data);
}
}
/**
* Finalizes audio processing by combining all received audio chunks,
* creating a Blob, and playing back the synthesized audio.
* If autoplay is blocked, a play button is created.
*/
finishAudioProcessing() {
if (!this.audioChunks.length) {
console.error("No audio received.");
return;
}
const audioBlob = new Blob(this.audioChunks, { type: "audio/mpeg" });
const audioUrl = URL.createObjectURL(audioBlob);
const audio = new Audio(audioUrl);
audio.play().catch(err => {
const playButton = document.createElement("button");
playButton.textContent = "Play TTS Audio";
document.body.appendChild(playButton);
playButton.onclick = () => {
audio.play();
playButton.remove();
};
});
// Close the WebSocket connection if it's still open.
if (this.ws && this.ws.readyState === WebSocket.OPEN) {
this.ws.close();
}
}
/**
* Initiates the entire speech synthesis process for the provided text.
* It selects the best voice, connects to the service, sends configuration and SSML requests,
* and ultimately plays the synthesized audio.
* @param {string} text - The text to be spoken.
*/
async speak(text) {
// A brief pause inserted before the text to help with smooth transitions.
const pause = ".. ... . . . . . ";
// Select the best voice based on preferences and available voices.
this.voice = await this.getBestVoice();
// Connect to the TTS service, send configuration, and then send the SSML request.
await this.connect();
await this.sendAudioConfig();
// The text is modified slightly for pacing (do not change this without testing).
await this.sendSSML(pause + text.replace(".", ". .").replace(",", ",,,,, "));
}
}
</script>
The Glue that calls the class and makes it all work
<script>
// Self-invoking async function to demonstrate how to use the EdgeTTS class.
// Modify this section to integrate TTS into your own application.
(async () => {
// Create an instance of the EdgeTTS class.
// This instance will handle fetching voices, establishing the WebSocket connection,
// sending SSML requests, and playing back the synthesized audio.
const tts = new EdgeTTS();
// Define the text you want to convert to speech.
// You can change this string to any text that you want the TTS system to speak.
const text = "The small, curious cat, with its soft, silky fur, carefully stepped onto the warm, cozy mat by the fireplace, stretching its legs and curling up comfortably as the golden glow of the fire flickered across the room.";
// Fetch the list of available voices from the EdgeTTS service.
// This step caches the voices in localStorage for faster retrieval later.
await tts.fetchVoices();
// Try to synthesize speech using the provided text.
// The speak() method will:
// 1. Select the best available voice (or use the default one).
// 2. Connect to the TTS WebSocket endpoint.
// 3. Send the necessary audio configuration.
// 4. Send an SSML request containing your text.
// 5. Receive audio data and play it back in the browser.
try {
console.log("Starting TTS...");
await tts.speak(text);
} catch (error) {
// If there is an error during the TTS process, log it to the console.
console.error("TTS Error:", error);
}
})();
</script>
The glue without comments, can you see clearly now?
<script src="js/drm.js"><!-- Include the DRM and EdgeTTS code --> </script>
<script>
(async () => {
const tts = new EdgeTTS();
const text = "The small, curious cat, with its soft, silky fur, carefully stepped onto the warm, cozy mat by the fireplace, stretching its legs and curling up comfortably as the golden glow of the fire flickered across the room.";
await tts.fetchVoices();
try {
console.log("Starting TTS...");
await tts.speak(text);
} catch (error) {
console.error("TTS Error:", error);
}
})();
</script>
It works directly in the browser using pure JavaScript—there is no need for Python or external plugins. It connects to the same API, sends SSML requests, and plays audio instantly.
Who needs Python when JavaScript rocks? The mystery of Edge-TTS now not quite unsolved, however one thing is clear: you can get high-quality speech synthesis directly in your browser, and no backend is needed.
Want to take it further? Clone the code, experiment, and tweak the voices. Just be mindful—Microsoft might change the API at any moment. Until then, enjoy the ride!
Here are the voices you can use, and it is as simple as a change to one line
Name Gender ContentCategories VoicePersonalities
--------------------------------- -------- --------------------- --------------------------------------
af-ZA-AdriNeural Female General Friendly, Positive
af-ZA-WillemNeural Male General Friendly, Positive
am-ET-AmehaNeural Male General Friendly, Positive
am-ET-MekdesNeural Female General Friendly, Positive
ar-AE-FatimaNeural Female General Friendly, Positive
ar-AE-HamdanNeural Male General Friendly, Positive
ar-BH-AliNeural Male General Friendly, Positive
ar-BH-LailaNeural Female General Friendly, Positive
ar-DZ-AminaNeural Female General Friendly, Positive
ar-DZ-IsmaelNeural Male General Friendly, Positive
ar-EG-SalmaNeural Female General Friendly, Positive
ar-EG-ShakirNeural Male General Friendly, Positive
ar-IQ-BasselNeural Male General Friendly, Positive
ar-IQ-RanaNeural Female General Friendly, Positive
ar-JO-SanaNeural Female General Friendly, Positive
ar-JO-TaimNeural Male General Friendly, Positive
ar-KW-FahedNeural Male General Friendly, Positive
ar-KW-NouraNeural Female General Friendly, Positive
ar-LB-LaylaNeural Female General Friendly, Positive
ar-LB-RamiNeural Male General Friendly, Positive
ar-LY-ImanNeural Female General Friendly, Positive
ar-LY-OmarNeural Male General Friendly, Positive
ar-MA-JamalNeural Male General Friendly, Positive
ar-MA-MounaNeural Female General Friendly, Positive
ar-OM-AbdullahNeural Male General Friendly, Positive
ar-OM-AyshaNeural Female General Friendly, Positive
ar-QA-AmalNeural Female General Friendly, Positive
ar-QA-MoazNeural Male General Friendly, Positive
ar-SA-HamedNeural Male General Friendly, Positive
ar-SA-ZariyahNeural Female General Friendly, Positive
ar-SY-AmanyNeural Female General Friendly, Positive
ar-SY-LaithNeural Male General Friendly, Positive
ar-TN-HediNeural Male General Friendly, Positive
ar-TN-ReemNeural Female General Friendly, Positive
ar-YE-MaryamNeural Female General Friendly, Positive
ar-YE-SalehNeural Male General Friendly, Positive
az-AZ-BabekNeural Male General Friendly, Positive
az-AZ-BanuNeural Female General Friendly, Positive
bg-BG-BorislavNeural Male General Friendly, Positive
bg-BG-KalinaNeural Female General Friendly, Positive
bn-BD-NabanitaNeural Female General Friendly, Positive
bn-BD-PradeepNeural Male General Friendly, Positive
bn-IN-BashkarNeural Male General Friendly, Positive
bn-IN-TanishaaNeural Female General Friendly, Positive
bs-BA-GoranNeural Male General Friendly, Positive
bs-BA-VesnaNeural Female General Friendly, Positive
ca-ES-EnricNeural Male General Friendly, Positive
ca-ES-JoanaNeural Female General Friendly, Positive
cs-CZ-AntoninNeural Male General Friendly, Positive
cs-CZ-VlastaNeural Female General Friendly, Positive
cy-GB-AledNeural Male General Friendly, Positive
cy-GB-NiaNeural Female General Friendly, Positive
da-DK-ChristelNeural Female General Friendly, Positive
da-DK-JeppeNeural Male General Friendly, Positive
de-AT-IngridNeural Female General Friendly, Positive
de-AT-JonasNeural Male General Friendly, Positive
de-CH-JanNeural Male General Friendly, Positive
de-CH-LeniNeural Female General Friendly, Positive
de-DE-AmalaNeural Female General Friendly, Positive
de-DE-ConradNeural Male General Friendly, Positive
de-DE-FlorianMultilingualNeural Male General Friendly, Positive
de-DE-KatjaNeural Female General Friendly, Positive
de-DE-KillianNeural Male General Friendly, Positive
de-DE-SeraphinaMultilingualNeural Female General Friendly, Positive
el-GR-AthinaNeural Female General Friendly, Positive
el-GR-NestorasNeural Male General Friendly, Positive
en-AU-NatashaNeural Female General Friendly, Positive
en-AU-WilliamNeural Male General Friendly, Positive
en-CA-ClaraNeural Female General Friendly, Positive
en-CA-LiamNeural Male General Friendly, Positive
en-GB-LibbyNeural Female General Friendly, Positive
en-GB-MaisieNeural Female General Friendly, Positive
en-GB-RyanNeural Male General Friendly, Positive
en-GB-SoniaNeural Female General Friendly, Positive
en-GB-ThomasNeural Male General Friendly, Positive
en-HK-SamNeural Male General Friendly, Positive
en-HK-YanNeural Female General Friendly, Positive
en-IE-ConnorNeural Male General Friendly, Positive
en-IE-EmilyNeural Female General Friendly, Positive
en-IN-NeerjaExpressiveNeural Female General Friendly, Positive
en-IN-NeerjaNeural Female General Friendly, Positive
en-IN-PrabhatNeural Male General Friendly, Positive
en-KE-AsiliaNeural Female General Friendly, Positive
en-KE-ChilembaNeural Male General Friendly, Positive
en-NG-AbeoNeural Male General Friendly, Positive
en-NG-EzinneNeural Female General Friendly, Positive
en-NZ-MitchellNeural Male General Friendly, Positive
en-NZ-MollyNeural Female General Friendly, Positive
en-PH-JamesNeural Male General Friendly, Positive
en-PH-RosaNeural Female General Friendly, Positive
en-SG-LunaNeural Female General Friendly, Positive
en-SG-WayneNeural Male General Friendly, Positive
en-TZ-ElimuNeural Male General Friendly, Positive
en-TZ-ImaniNeural Female General Friendly, Positive
en-US-AnaNeural Female Cartoon, Conversation Cute
en-US-AndrewMultilingualNeural Male Conversation, Copilot Warm, Confident, Authentic, Honest
en-US-AndrewNeural Male Conversation, Copilot Warm, Confident, Authentic, Honest
en-US-AriaNeural Female News, Novel Positive, Confident
en-US-AvaMultilingualNeural Female Conversation, Copilot Expressive, Caring, Pleasant, Friendly
en-US-AvaNeural Female Conversation, Copilot Expressive, Caring, Pleasant, Friendly
en-US-BrianMultilingualNeural Male Conversation, Copilot Approachable, Casual, Sincere
en-US-BrianNeural Male Conversation, Copilot Approachable, Casual, Sincere
en-US-ChristopherNeural Male News, Novel Reliable, Authority
en-US-EmmaMultilingualNeural Female Conversation, Copilot Cheerful, Clear, Conversational
en-US-EmmaNeural Female Conversation, Copilot Cheerful, Clear, Conversational
en-US-EricNeural Male News, Novel Rational
en-US-GuyNeural Male News, Novel Passion
en-US-JennyNeural Female General Friendly, Considerate, Comfort
en-US-MichelleNeural Female News, Novel Friendly, Pleasant
en-US-RogerNeural Male News, Novel Lively
en-US-SteffanNeural Male News, Novel Rational
en-ZA-LeahNeural Female General Friendly, Positive
en-ZA-LukeNeural Male General Friendly, Positive
es-AR-ElenaNeural Female General Friendly, Positive
es-AR-TomasNeural Male General Friendly, Positive
es-BO-MarceloNeural Male General Friendly, Positive
es-BO-SofiaNeural Female General Friendly, Positive
es-CL-CatalinaNeural Female General Friendly, Positive
es-CL-LorenzoNeural Male General Friendly, Positive
es-CO-GonzaloNeural Male General Friendly, Positive
es-CO-SalomeNeural Female General Friendly, Positive
es-CR-JuanNeural Male General Friendly, Positive
es-CR-MariaNeural Female General Friendly, Positive
es-CU-BelkysNeural Female General Friendly, Positive
es-CU-ManuelNeural Male General Friendly, Positive
es-DO-EmilioNeural Male General Friendly, Positive
es-DO-RamonaNeural Female General Friendly, Positive
es-EC-AndreaNeural Female General Friendly, Positive
es-EC-LuisNeural Male General Friendly, Positive
es-ES-AlvaroNeural Male General Friendly, Positive
es-ES-ElviraNeural Female General Friendly, Positive
es-ES-XimenaNeural Female General Friendly, Positive
es-GQ-JavierNeural Male General Friendly, Positive
es-GQ-TeresaNeural Female General Friendly, Positive
es-GT-AndresNeural Male General Friendly, Positive
es-GT-MartaNeural Female General Friendly, Positive
es-HN-CarlosNeural Male General Friendly, Positive
es-HN-KarlaNeural Female General Friendly, Positive
es-MX-DaliaNeural Female General Friendly, Positive
es-MX-JorgeNeural Male General Friendly, Positive
es-NI-FedericoNeural Male General Friendly, Positive
es-NI-YolandaNeural Female General Friendly, Positive
es-PA-MargaritaNeural Female General Friendly, Positive
es-PA-RobertoNeural Male General Friendly, Positive
es-PE-AlexNeural Male General Friendly, Positive
es-PE-CamilaNeural Female General Friendly, Positive
es-PR-KarinaNeural Female General Friendly, Positive
es-PR-VictorNeural Male General Friendly, Positive
es-PY-MarioNeural Male General Friendly, Positive
es-PY-TaniaNeural Female General Friendly, Positive
es-SV-LorenaNeural Female General Friendly, Positive
es-SV-RodrigoNeural Male General Friendly, Positive
es-US-AlonsoNeural Male General Friendly, Positive
es-US-PalomaNeural Female General Friendly, Positive
es-UY-MateoNeural Male General Friendly, Positive
es-UY-ValentinaNeural Female General Friendly, Positive
es-VE-PaolaNeural Female General Friendly, Positive
es-VE-SebastianNeural Male General Friendly, Positive
et-EE-AnuNeural Female General Friendly, Positive
et-EE-KertNeural Male General Friendly, Positive
fa-IR-DilaraNeural Female General Friendly, Positive
fa-IR-FaridNeural Male General Friendly, Positive
fi-FI-HarriNeural Male General Friendly, Positive
fi-FI-NooraNeural Female General Friendly, Positive
fil-PH-AngeloNeural Male General Friendly, Positive
fil-PH-BlessicaNeural Female General Friendly, Positive
fr-BE-CharlineNeural Female General Friendly, Positive
fr-BE-GerardNeural Male General Friendly, Positive
fr-CA-AntoineNeural Male General Friendly, Positive
fr-CA-JeanNeural Male General Friendly, Positive
fr-CA-SylvieNeural Female General Friendly, Positive
fr-CA-ThierryNeural Male General Friendly, Positive
fr-CH-ArianeNeural Female General Friendly, Positive
fr-CH-FabriceNeural Male General Friendly, Positive
fr-FR-DeniseNeural Female General Friendly, Positive
fr-FR-EloiseNeural Female General Friendly, Positive
fr-FR-HenriNeural Male General Friendly, Positive
fr-FR-RemyMultilingualNeural Male General Friendly, Positive
fr-FR-VivienneMultilingualNeural Female General Friendly, Positive
ga-IE-ColmNeural Male General Friendly, Positive
ga-IE-OrlaNeural Female General Friendly, Positive
gl-ES-RoiNeural Male General Friendly, Positive
gl-ES-SabelaNeural Female General Friendly, Positive
gu-IN-DhwaniNeural Female General Friendly, Positive
gu-IN-NiranjanNeural Male General Friendly, Positive
he-IL-AvriNeural Male General Friendly, Positive
he-IL-HilaNeural Female General Friendly, Positive
hi-IN-MadhurNeural Male General Friendly, Positive
hi-IN-SwaraNeural Female General Friendly, Positive
hr-HR-GabrijelaNeural Female General Friendly, Positive
hr-HR-SreckoNeural Male General Friendly, Positive
hu-HU-NoemiNeural Female General Friendly, Positive
hu-HU-TamasNeural Male General Friendly, Positive
id-ID-ArdiNeural Male General Friendly, Positive
id-ID-GadisNeural Female General Friendly, Positive
is-IS-GudrunNeural Female General Friendly, Positive
is-IS-GunnarNeural Male General Friendly, Positive
it-IT-DiegoNeural Male General Friendly, Positive
it-IT-ElsaNeural Female General Friendly, Positive
it-IT-GiuseppeMultilingualNeural Male General Friendly, Positive
it-IT-IsabellaNeural Female General Friendly, Positive
iu-Cans-CA-SiqiniqNeural Female General Friendly, Positive
iu-Cans-CA-TaqqiqNeural Male General Friendly, Positive
iu-Latn-CA-SiqiniqNeural Female General Friendly, Positive
iu-Latn-CA-TaqqiqNeural Male General Friendly, Positive
ja-JP-KeitaNeural Male General Friendly, Positive
ja-JP-NanamiNeural Female General Friendly, Positive
jv-ID-DimasNeural Male General Friendly, Positive
jv-ID-SitiNeural Female General Friendly, Positive
ka-GE-EkaNeural Female General Friendly, Positive
ka-GE-GiorgiNeural Male General Friendly, Positive
kk-KZ-AigulNeural Female General Friendly, Positive
kk-KZ-DauletNeural Male General Friendly, Positive
km-KH-PisethNeural Male General Friendly, Positive
km-KH-SreymomNeural Female General Friendly, Positive
kn-IN-GaganNeural Male General Friendly, Positive
kn-IN-SapnaNeural Female General Friendly, Positive
ko-KR-HyunsuMultilingualNeural Male General Friendly, Positive
ko-KR-InJoonNeural Male General Friendly, Positive
ko-KR-SunHiNeural Female General Friendly, Positive
lo-LA-ChanthavongNeural Male General Friendly, Positive
lo-LA-KeomanyNeural Female General Friendly, Positive
lt-LT-LeonasNeural Male General Friendly, Positive
lt-LT-OnaNeural Female General Friendly, Positive
lv-LV-EveritaNeural Female General Friendly, Positive
lv-LV-NilsNeural Male General Friendly, Positive
mk-MK-AleksandarNeural Male General Friendly, Positive
mk-MK-MarijaNeural Female General Friendly, Positive
ml-IN-MidhunNeural Male General Friendly, Positive
ml-IN-SobhanaNeural Female General Friendly, Positive
mn-MN-BataaNeural Male General Friendly, Positive
mn-MN-YesuiNeural Female General Friendly, Positive
mr-IN-AarohiNeural Female General Friendly, Positive
mr-IN-ManoharNeural Male General Friendly, Positive
ms-MY-OsmanNeural Male General Friendly, Positive
ms-MY-YasminNeural Female General Friendly, Positive
mt-MT-GraceNeural Female General Friendly, Positive
mt-MT-JosephNeural Male General Friendly, Positive
my-MM-NilarNeural Female General Friendly, Positive
my-MM-ThihaNeural Male General Friendly, Positive
nb-NO-FinnNeural Male General Friendly, Positive
nb-NO-PernilleNeural Female General Friendly, Positive
ne-NP-HemkalaNeural Female General Friendly, Positive
ne-NP-SagarNeural Male General Friendly, Positive
nl-BE-ArnaudNeural Male General Friendly, Positive
nl-BE-DenaNeural Female General Friendly, Positive
nl-NL-ColetteNeural Female General Friendly, Positive
nl-NL-FennaNeural Female General Friendly, Positive
nl-NL-MaartenNeural Male General Friendly, Positive
pl-PL-MarekNeural Male General Friendly, Positive
pl-PL-ZofiaNeural Female General Friendly, Positive
ps-AF-GulNawazNeural Male General Friendly, Positive
ps-AF-LatifaNeural Female General Friendly, Positive
pt-BR-AntonioNeural Male General Friendly, Positive
pt-BR-FranciscaNeural Female General Friendly, Positive
pt-BR-ThalitaMultilingualNeural Female General Friendly, Positive
pt-PT-DuarteNeural Male General Friendly, Positive
pt-PT-RaquelNeural Female General Friendly, Positive
ro-RO-AlinaNeural Female General Friendly, Positive
ro-RO-EmilNeural Male General Friendly, Positive
ru-RU-DmitryNeural Male General Friendly, Positive
ru-RU-SvetlanaNeural Female General Friendly, Positive
si-LK-SameeraNeural Male General Friendly, Positive
si-LK-ThiliniNeural Female General Friendly, Positive
sk-SK-LukasNeural Male General Friendly, Positive
sk-SK-ViktoriaNeural Female General Friendly, Positive
sl-SI-PetraNeural Female General Friendly, Positive
sl-SI-RokNeural Male General Friendly, Positive
so-SO-MuuseNeural Male General Friendly, Positive
so-SO-UbaxNeural Female General Friendly, Positive
sq-AL-AnilaNeural Female General Friendly, Positive
sq-AL-IlirNeural Male General Friendly, Positive
sr-RS-NicholasNeural Male General Friendly, Positive
sr-RS-SophieNeural Female General Friendly, Positive
su-ID-JajangNeural Male General Friendly, Positive
su-ID-TutiNeural Female General Friendly, Positive
sv-SE-MattiasNeural Male General Friendly, Positive
sv-SE-SofieNeural Female General Friendly, Positive
sw-KE-RafikiNeural Male General Friendly, Positive
sw-KE-ZuriNeural Female General Friendly, Positive
sw-TZ-DaudiNeural Male General Friendly, Positive
sw-TZ-RehemaNeural Female General Friendly, Positive
ta-IN-PallaviNeural Female General Friendly, Positive
ta-IN-ValluvarNeural Male General Friendly, Positive
ta-LK-KumarNeural Male General Friendly, Positive
ta-LK-SaranyaNeural Female General Friendly, Positive
ta-MY-KaniNeural Female General Friendly, Positive
ta-MY-SuryaNeural Male General Friendly, Positive
ta-SG-AnbuNeural Male General Friendly, Positive
ta-SG-VenbaNeural Female General Friendly, Positive
te-IN-MohanNeural Male General Friendly, Positive
te-IN-ShrutiNeural Female General Friendly, Positive
th-TH-NiwatNeural Male General Friendly, Positive
th-TH-PremwadeeNeural Female General Friendly, Positive
tr-TR-AhmetNeural Male General Friendly, Positive
tr-TR-EmelNeural Female General Friendly, Positive
uk-UA-OstapNeural Male General Friendly, Positive
uk-UA-PolinaNeural Female General Friendly, Positive
ur-IN-GulNeural Female General Friendly, Positive
ur-IN-SalmanNeural Male General Friendly, Positive
ur-PK-AsadNeural Male General Friendly, Positive
ur-PK-UzmaNeural Female General Friendly, Positive
uz-UZ-MadinaNeural Female General Friendly, Positive
uz-UZ-SardorNeural Male General Friendly, Positive
vi-VN-HoaiMyNeural Female General Friendly, Positive
vi-VN-NamMinhNeural Male General Friendly, Positive
zh-CN-XiaoxiaoNeural Female News, Novel Warm
zh-CN-XiaoyiNeural Female Cartoon, Novel Lively
zh-CN-YunjianNeural Male Sports, Novel Passion
zh-CN-YunxiNeural Male Novel Lively, Sunshine
zh-CN-YunxiaNeural Male Cartoon, Novel Cute
zh-CN-YunyangNeural Male News Professional, Reliable
zh-CN-liaoning-XiaobeiNeural Female Dialect Humorous
zh-CN-shaanxi-XiaoniNeural Female Dialect Bright
zh-HK-HiuGaaiNeural Female General Friendly, Positive
zh-HK-HiuMaanNeural Female General Friendly, Positive
zh-HK-WanLungNeural Male General Friendly, Positive
zh-TW-HsiaoChenNeural Female General Friendly, Positive
zh-TW-HsiaoYuNeural Female General Friendly, Positive
zh-TW-YunJheNeural Male General Friendly, Positive
zu-ZA-ThandoNeural Female General Friendly, Positive
zu-ZA-ThembaNeural Male General Friendly, Positive
Now Over to You
Tried it? Loved it? Do you have any thoughts about the legality of Edge-TTS? Drop a comment below! 🚀🐱🔥